# Classes - JS
Classes are a tool that developers use to quickly produce similar objects.
Create a template of an object -› reduce duplicate code and debugging time.
class Dog {
constructor(name) {
this._name = name;
this._behavior = 0;
}
get name() {
return this._name;
}
get behavior() {
return this._behavior;
}
incrementBehavior() {
this._behavior++;
}
}
# Constructor
JavaScript calls the constructor()
method every time it creates a new instance of a class.
class Dog {
constructor(name) {
this.name = name;
this.behavior = 0;
}
}
Dog
is the name of our class. By convention, we capitalize and CamelCase class names.- Inside of the
constructor()
method, we use thethis
keyword -› refers to an instance of that class.
# Instance
An instance is an object that contains the property names and methods of a class, but with unique property values.
Use new
- Javascript calls a constructor method when we create a new instance of a class.
class Dog {
constructor(name) {
this.name = name;
this.behavior = 0;
}
}
const halley = new Dog('Halley'); // Create new Dog instance
console.log(halley.name); // Log the name value saved to halley
// Output: 'Halley'
# Methods
- Class method and getter syntax is the same as it is for objects except you don't include commas between methods.
class Dog {
constructor(name) {
this._name = name;
this._behavior = 0;
}
get name() {
return this._name;
}
get behavior() {
return this._behavior;
}
incrementBehavior() {
this._behavior++;
}
}
- use getter methods for
name
andbehavior
- prepended the property names with underscores (
_name
and_behavior
), which indicate these properties should not be accessed directly.
# Inheritance
Create a parent class with properties and methods that we can extend to child classes.
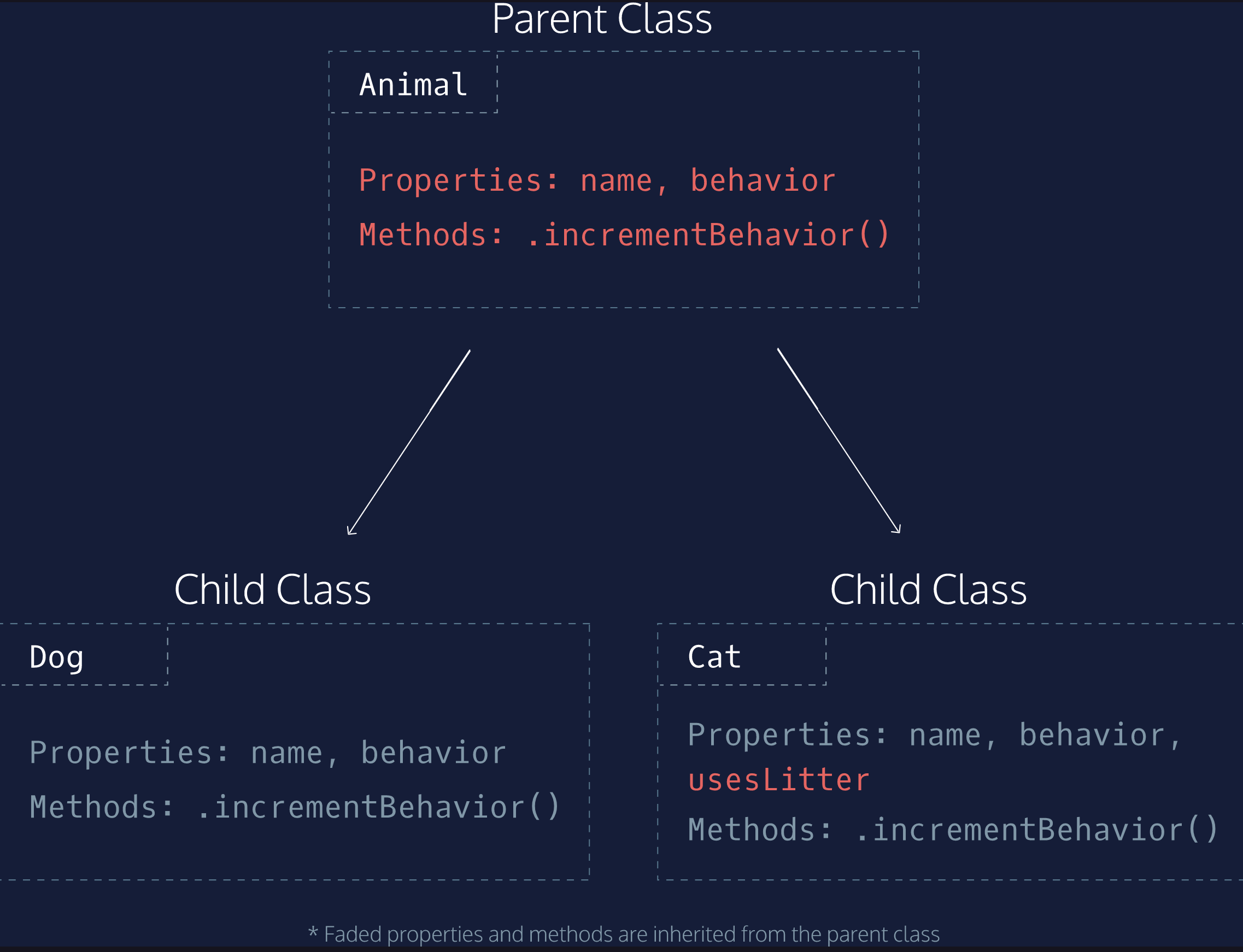
When multiple classes share properties or methods, they become candidates for inheritance —
# Parent Class
With inheritance, you can create a parent class (also known as a superclass) with properties and methods that multiple child classes (also known as subclasses) share.
class Animal {
constructor(name) {
this._name = name;
this._behavior = 0;
}
get name() {
return this._name;
}
get behavior() {
return this._behavior;
}
incrementBehavior() {
this._behavior++;
}
}
In the example above, the Animal
class contains the properties and methods that the Cat
and Dog
classes share (name
, behavior
, .incrementBehavior()
).
# Subclass
- Use the
extends
keyword to create a subclass. - The
super
keyword calls theconstructor()
of a parent class.
class Cat extends Animal {
constructor(name, usesLitter) {
super(name);
this._usesLitter = usesLitter;
}
}
Create a new Cat
instance:
const luluCat = new Cat('Lulu', false);
- The
extends
keyword makes the methods of the animal class available inside the cat class. - The constructor, called when you create a new
Cat
object, accepts two arguments,name
andusesLitter
. - The
super
keyword calls the constructor of the parent class. _usesLitter
is a new property that is unique to theCat
class, so we set it in theCat
constructor.
In a constructor()
, you must always call the super
method before you can use the this
keyword - otherwise, JavaScript will throw a reference error.
Best practice: call super
on the first line of subclass constructors.
# Subclass Methods:
All of the parent methods are available to the child class.
Because luluCat
has access to the name
getter, the code below logs 'Lulu'
to the console.
console.log(luluCat.name);
Additionally, child classes can contain their own properties, getters, setters, and methods.
class Cat extends Animal {
constructor(name, usesLitter) {
super(name);
this._usesLitter = usesLitter;
}
get usesLitter() {
return this._usesLitter;
}
}
# Static Methods
Static methods are called on the class, but not on instances of the class.
Take the
Date
class, for example — you can both createDate
instances to represent whatever date you want, and call static methods, likeDate.now()
which returns the current date. The.now()
method is static, so you can call it directly from the class, but not from an instance of the class.
Use the static
keyword to create a static method called generateName
class Animal {
constructor(name) {
this._name = name;
this._behavior = 0;
}
static generateName() {
const names = ['Angel', 'Spike', 'Buffy', 'Willow', 'Tara'];
const randomNumber = Math.floor(Math.random() * 5);
return names[randomNumber];
}
}
In the example above, the static
method called .generateName()
returns a random name when it’s called.
Call the .generateName()
method with the following syntax:
console.log(Animal.generateName()); // returns a name
You cannot access the .generateName()
method from instances of the Animal
class or instances of its subclasses.
# Simple constructor function
capitalized!
function HouseKeeper(name, age, hasWorkPermit) {
this.name = name;
this.age = age;
this.hasWorkPermit = hasWorkPermit;
}
const houseKeeper1 = new HouseKeeper('Tom', 29, true);
# methods
function HouseKeeper(name, age, hasWorkPermit) {
this.name = name;
this.age = age;
this.hasWorkPermit = hasWorkPermit;
this.clean = function() {
alert('cleaning in progress');
};
}